In the dynamic world of JavaScript, coding conventions and rules are essential for maintaining code readability, consistency, and collaboration within teams. Using coding standards streamlines debugging and reduces errors. For beginners and students, understanding these standards is crucial, as it ensures that code is not only functional, but also clean and easy to comprehend for others.
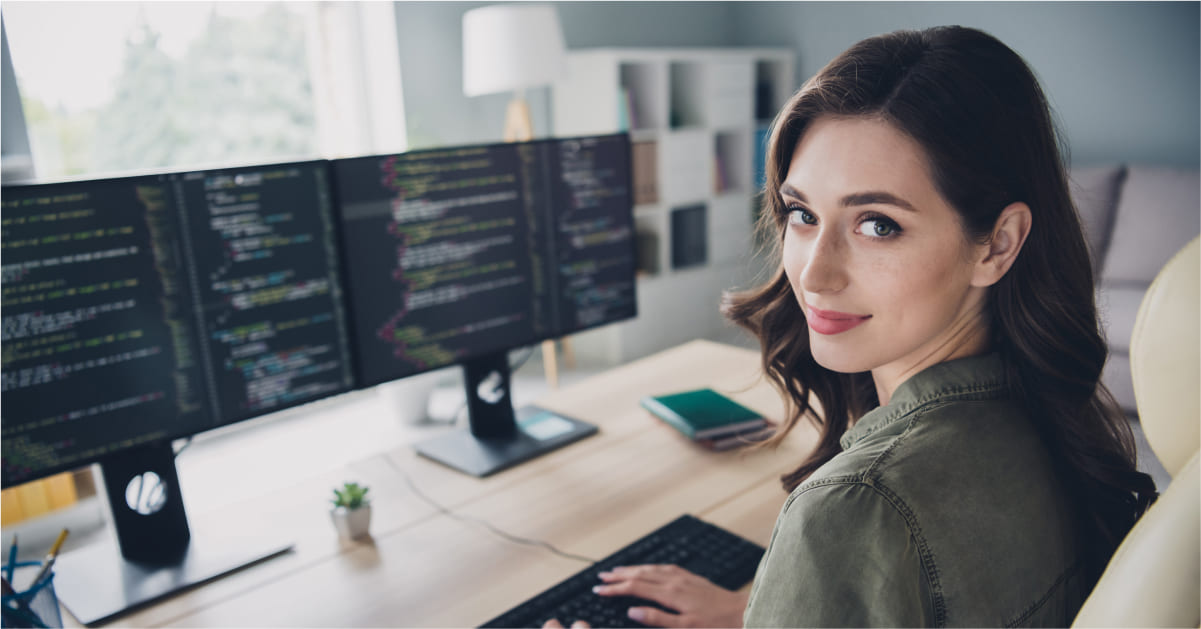
This is the first article in a series on the topic of coding standards. We’ll dive into the importance of commenting in JavaScript code and how to do it right. Let’s go!
Why are coding standards important?
The long-term value of software is directly proportional to the quality of its codebase. Over time, your code will be handled by many developers. If your code is clear and well-structured, it is less likely to break when modified in the future.
This is where coding standards come in to help reduce the brittleness of programs and make your code robust and resilient.
Overview of JavaScript coding standards
JavaScript coding standards encompass various aspects of coding, such as:
- Naming conventions
- Code formatting
- Commenting practices
- Error handling
Following these standards ensures that your code is consistent and easy to understand.
Commenting in JavaScript
Comments in JavaScript play a crucial role in making your code understandable and maintainable.
For beginners and students, learning how to effectively comment code is essential. Comments provide context, explain complex logic, and make it easier for others (and your future self) to navigate and modify the code.
Importance of commenting
Other developers will use your comments to understand your code without having to decipher it from scratch. Properly commented code is easier to debug, maintain, and extend.
Comments can explain why certain decisions were made, what specific parts of the code do, and any assumptions or special cases handled.
Guidelines for normal comments
Multiple line comments: For comments that stretch over multiple lines, use the multiple line comment format (/* comment */). This format is easier to manage and extend if the comment grows.
/* * This is a multiple line comment. * It can span multiple lines easily. */
Single line comments: For short, one-line comments, you can use either the multiple line format or the single line format (// comment). Consistency is key, so choose one style and stick with it for better readability.
// This is a single line comment.
Documentation comments with JSDoc
Every function or class should have a documentation comment that tells other programmers everything they need to know to use it.
For clear and effective code documentation, every parameter’s purpose, the expected input, and the output must be included in the comments. It’s also essential to document how the function behaves during error conditions and what those conditions are. Developers should not need to examine the source code to use the function with confidence.
Any code that is complex, obscure, or not immediately clear should be thoroughly commented. It’s crucial to document any assumptions or preconditions required for the code to function correctly. This helps ensure that any software developer can understand any part of the application quickly and efficiently.
JSDoc format is a standard for documenting JavaScript code. Make sure that all documentation comments are in JSDoc format. For more details, read this article.
Here’s what you should include in the comments:
- Description of the function or class
- Parameters – Document each parameter with the @param tag.
- Return Value – Document the return value with the @return tag.
- Exceptions – Use the @throws tag to document any exceptions.
And it should look like this:
/* * Adds two numbers together. * @param {number} a - The first number. * @param {number} b - The second number. * @return {number} The sum of the two numbers. */ function add(a, b) { return a + b; }
Commenting checklist
To ensure your comments are effective, follow this checklist:
- Clarity – Can someone pick up the code and immediately start to understand it?
- Intent – Do comments explain the code’s intent rather than just repeating the code?
- Moderation – Has tricky code been rewritten rather than over-commented?
- Update – Are comments up-to-date with the code?
- Focus – Do comments focus on why the code is written a certain way rather than how it works?
- Comprehensive – Are the following elements commented on: input and output data, interface assumptions, limitations, sources of algorithms, behavior in error conditions, and so on?
- Others – Are magic numbers replaced with named constants rather than just documented? Are the ranges of values commented?
If you answered Yes to all these questions, you’re ready for the next step 🙂
Naming conventions in JavaScript
Naming your variables, functions, and classes properly is also key to writing clean and understandable JavaScript code.
Some case styles are common in computer programming because they improve readability and organization. Such case conventions provide a consistent style within codebases, reducing confusion and making it easier for teams to collaborate.
Let’s go over some general naming conventions.
Using Camel case
Camel case is a case style where the variable or function names are combined and only the second letter is capitalized. This style can be used for more purposes, like:
- Multi-world variables: e.g, authTokens
- Function names: e.g., getRegistered
- Function parameters: e.g., register(systemName, messageObject, registerInfo)
An example would look like this:
// Camel Case let authTokens = []; function getRegistered() {}
Some individuals and even some organizations use the term Camel case exclusively to refer to lower camel case, while referring to upper Camel case as Pascal case.
Using Pascal case
Pascal case is a casing style in which the words for a class or type name are joined together and the first letter of each is capitalized. This style should be used for:
- ‘enum like’ constants, like KernelSystem, KernelState. Please always use singular!
- Class names: e.g. UILRouter
- Default imports: e.g. import UILValidations from “./uil-validations.js”.
An example would look like this:
// Pascal Case class UILRouter {} import UILValidations from "./uil-validations.js";
Proper naming conventions prevent conflicts or misunderstandings about variable purpose and scope. And the perfect example here is differentiating between UserDetails (class) vs. userDetails (variable).
Using uppercase
The uppercase is usually used for module level constants, like AUTH_TOKEN_REGEXP.
An example would look like this:
// Upper Case const AUTH_TOKEN_REGEXP = /auth_token/;
First step, mission accomplished
Understanding JavaScript coding standards is the first step towards becoming a proficient programmer. By adhering to these standards, you ensure that your code is clean, readable, and maintainable.
Effective commenting is an integral part of writing clean and maintainable JavaScript code. By adhering to these guidelines and using the JSDoc format, you can make your code more understandable for yourself and others. Additionally, adopting consistent naming conventions will enhance your code’s readability and maintainability.
We have more JavaScript articles coming, but until then, you can read about closures in JavaScript and how to easily debug compiled JavaScript code.
In the next article of this series, we’ll dive into how to write clear and concise conditional statements in JavaScript. Furthermore, we’ll explore the use of control structures in JavaScript. Stay tuned!
Post A Reply